[패스트캠퍼스 수강 후기] 올인원 패키지 : 유니티 포트폴리오 완성 100% 환급 챌린지 35회차 미션 시작합니다.
04. 배틀로얄 - 15, 16 번을 진행합니다.
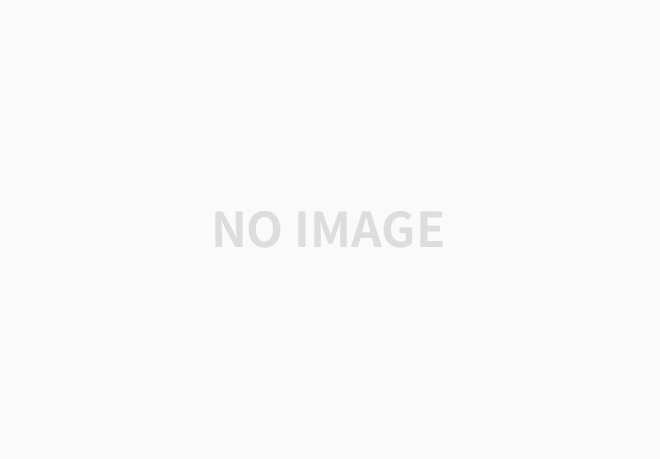
BaseData::GetDataCount() 함수의 오류가 있어서 버그를 수정한 내용입니다.
위와 같이 수정하고 저번시간의 EffectTool Window를 실행해 보면 기본적인 구동이 되는 것을 확인할 수 있습니다.
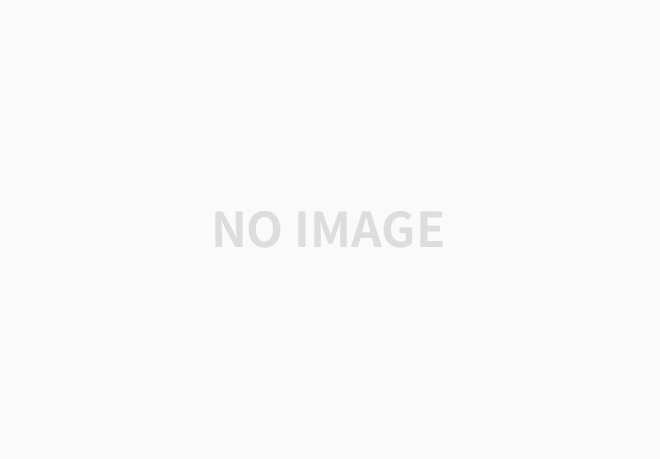
ADD를 클릭하여 Item을 하나 더 추가하고.. 이름을 설정하고 Prefabs/Effects에 준비된 Effect GameObject를 마우스클릭으로 드래그하여 이팩트에 가져다 놓으면 연결되어 동작하는 것도 확인할 수 있습니다.
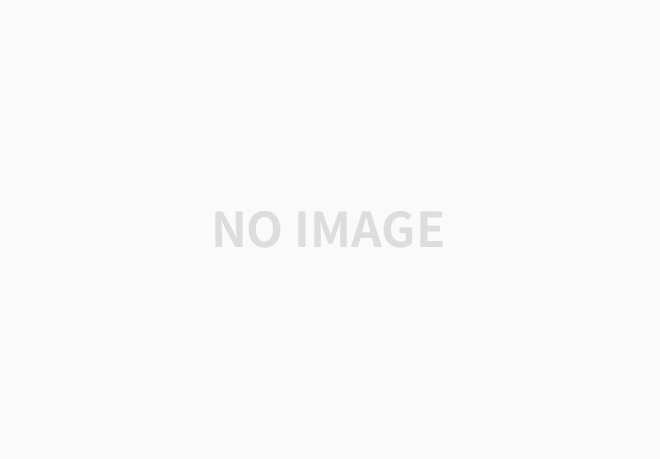
추가한 이후 [SAVE]를 클릭하면 effectData와 EffectList에 영향을 주게 됩니다.
우선 effectData 파일을 더블클릭하여 열어봅니다.
다음과 같이 한 줄로 구성된 xml 파일의 내용을 볼 수 있습니다.
이렇게 보면 좀 불편한대요..
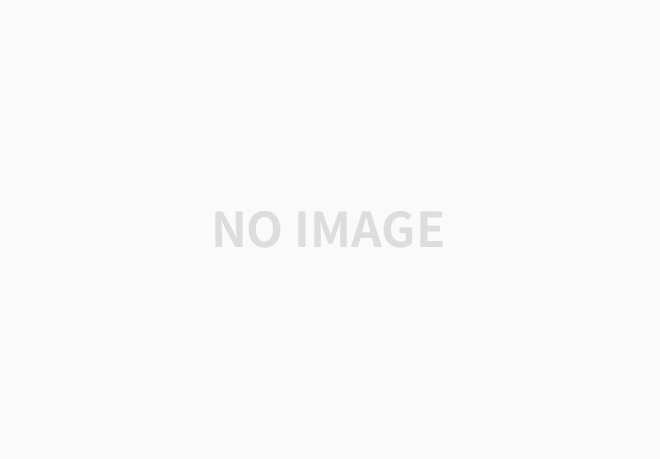
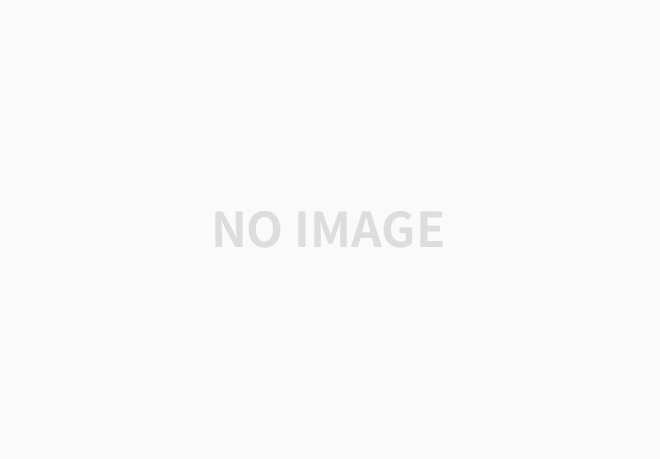
이렇게 Excel 문서에서 열어서 보면 좀더 구성이 잘된 모양의 동일한 effectData xml 파일의 내용을 볼 수가 있습니다.
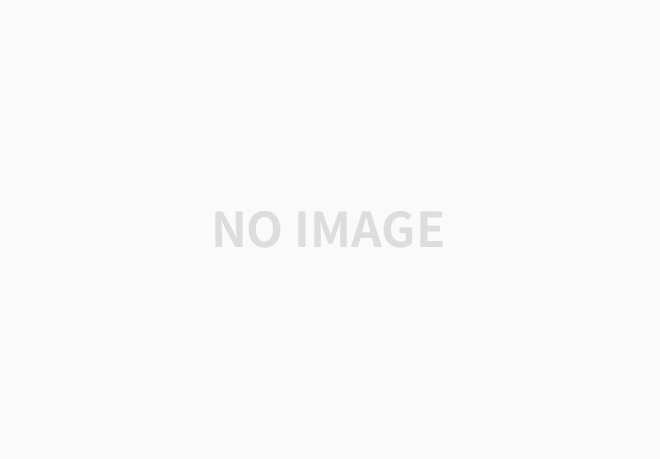
또한 EffectList 파일을 더블클릭하여 열어보면 EffectList라는 enum도 Tool에서 추가한 아이템 내용대로 업데이트된 것을 확인할 수가 있습니다.
이렇게 수행되도록 만든 코드가 CreateEnumStructure()라는 함수였고 이것이 수행된 결과인 것입니다.
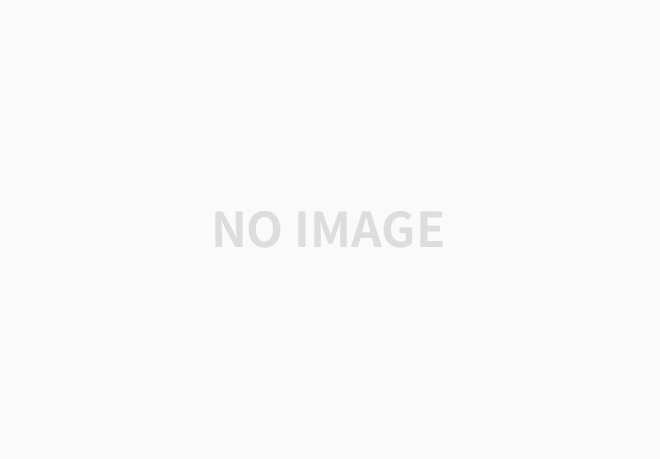
이제 Manager 폴더 아래에 DataManager와 EffectManager 2개의 스크립트를 준비합니다.
우선 DataManager부터 작성을 시작합니다.
public class DataManager : MonoBehaviour
{
private static EffectData effectData = null;
void Start() {
if (effectData == null) {
effectData = ScriptableObject.CreateInstance<EffectData>();
effectData.LoadData();
}
}
public static EffectData EffectData() {
if (effectData == null) {
effectData = ScriptableObject.CreateInstance<EffectData>();
effectData.LoadData();
}
return effectData;
}
}
이제 EffectManager를 작성합니다.
public class EffectManager : SingletonMonoBehaviour<EffectManager>
{
private Transform effectRoot = null;
void Start() {
effectRoot = new GameObject("EffectRoot").transform;
effectRoot.SetParent(transform);
}
//## 핵심 코드
public GameObject EffectOneShot(int index, Vector3 position) {
EffectClip clip = DataManager.EffectData().GetClip(index);
GameObject effectInstance = clip.Instantiate(position);
effectInstance.SetActive(true);
return effectInstance;
}
}
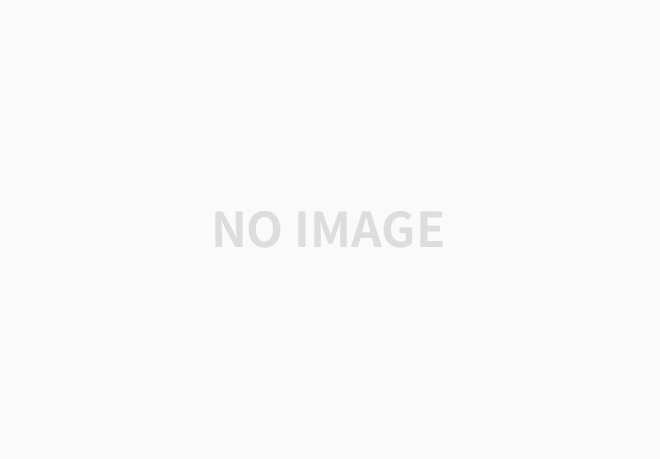
SingletonMonoBehaviour의 소스는 위와 같으며 프로젝트에 기본 포함하여 배포되었습니다.
위와같이 하여 EffectTool이 끝난거라고 합니다.. 무언가 Unity에서 확인해보고 구동하는 것이 있을거라 생각했는데 아니었네요 ㅎㅎ
바로 SoundTool 제작으로 넘어갑니다..
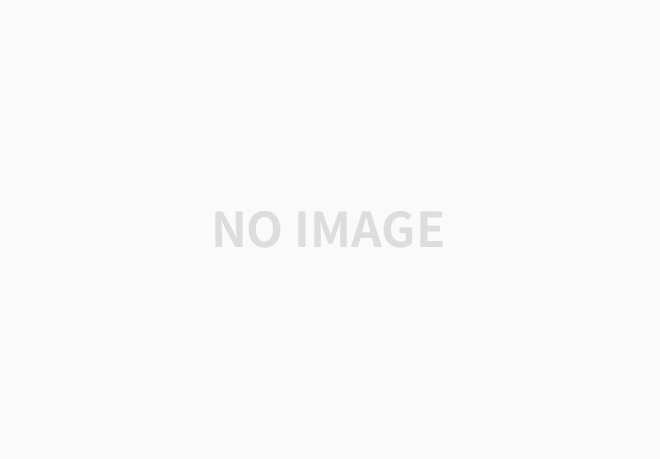
SoundClip 스크립트를 만들고 작성을 시작합니다 ^^; SoundTool은 EffectTool과 핵심적인 기능은 동일한데 Sound의 특성상 Loop 기능이나 CheckPoint 및 FadeOut등의 효과 등의 처리가 많아서 데이터가 많기 때문에 조금 복잡하다는 것만 다르다고 보면 됩니다.
// 루프, 페이드 관련 속성, 오디오 클립 속성들.
public class SoundClip
{
public SoundPlayType playTpe = SoundPlayType.None;
public string clipName = string.Empty;
public string clipPath = string.Empty;
public float maxVolume = 1.0f;
public bool isLoop = false;
public float[] checkTime = new float[0];
public float[] setTime = new float[0];
public int realId = 0;
private AudioClip clip = null;
public int currentLoop = 0;
public float pitch = 1.0f;
public float dopplerLevel = 1.0f;
public AudioRolloffMode rolloffMode = AudioRolloffMode.Logarithmic;
public float minDistance = 10000.0f;
public float maxDistance = 50000.0f;
public float sparialBlend = 1.0f;
public float fadeTime1 = 0.0f;
public float fadeTime2 = 0.0f;
public Interpolate.Function interpolate_Func;
public bool isFadeIn = false;
public bool isFadeOut = false;
public SoundClip() { }
public SoundClip(string clipPath, string clipName) {
this.clipPath = clipPath;
this.clipName = clipName;
}
public void PreLoad() {
if (this.clip == null) {
string fullPath = this.clipPath + this.clipName;
this.clip = ResourceManager.Load(fullPath) as AudioClip;
}
}
public void AddLoop() {
checkTime = ArrayHelper.Add(0.0f, this.checkTime);
setTime = ArrayHelper.Add(0.0f, this.setTime);
}
public void RemoveLoop(int index) {
checkTime = ArrayHelper.Remove(index, this.checkTime);
setTime = ArrayHelper.Remove(index, this.setTime);
}
public AudioClip GetClip() {
if (clip == null) PreLoad();
if (clip == null && clipName != string.Empty) {
Debug.LogWarning($"Can not load audio clip Resource {clipName}");
return null;
}
return clip;
}
public void ReleaseClip() {
if (clip != null) clip = null;
}
public bool HasLoop() {
return checkTime.Length > 0;
}
public void NextLoop() {
currentLoop++;
if (currentLoop >= checkTime.Length) currentLoop = 0;
}
public void CheckLoop(AudioSource source) {
if (HasLoop() && source.time >= checkTime[currentLoop]) {
source.time = setTime[currentLoop];
NextLoop();
}
}
public void FadeIn(float time, Interpolate.EaseType easeType) {
isFadeOut = false;
fadeTime1 = 0.0f;
fadeTime2 = time;
interpolate_Func = Interpolate.Ease(easeType);
isFadeIn = true;
}
public void FadeOut(float time, Interpolate.EaseType easeType) {
isFadeIn = false;
fadeTime1 = 0.0f;
fadeTime2 = time;
interpolate_Func = Interpolate.Ease(easeType);
isFadeOut = true;
}
/// <summary>
/// 페이드인,아웃 효과 프로세스
/// </summary>
public void DoFade(float time, AudioSource audio) {
if (isFadeIn == true) {
fadeTime1 += time;
audio.volume = Interpolate.Ease(interpolate_Func, 0, maxVolume, fadeTime1, fadeTime2);
if (fadeTime1 >= fadeTime2) isFadeIn = false;
}
else if (isFadeOut == true) {
fadeTime1 += time;
audio.volume = Interpolate.Ease(interpolate_Func, maxVolume, 0 - maxVolume, fadeTime1, fadeTime2);
if (fadeTime1 >= fadeTime2) {
isFadeOut = false;
audio.Stop();
}
}
}
}
여기까지가 SoundClip 완성입니다. 다음시간부터는 SoundManager를 통하여 Clip을 관리하는 기능을 구현할 것입니다.
결국 SoundClip은 소리 재생인데 게임 상황상 여러가지 옵션이 많다보니 함수를 많이 구현하게 된 것입니다.
Easing functions에 대해서는 구글에서 검색한 이미지를 하나 추가합니다.
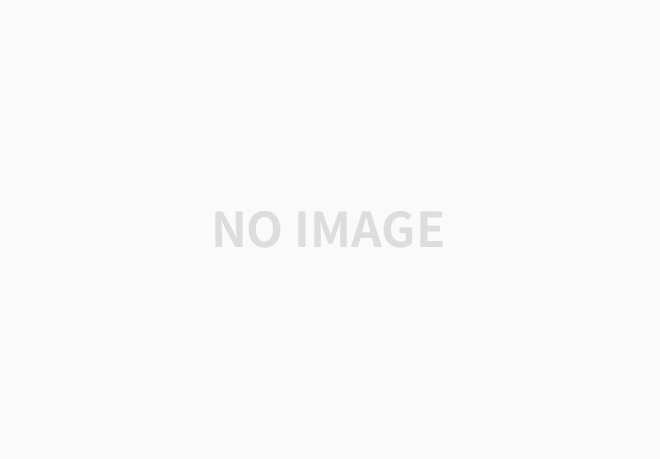
<위의 코드들은 제가 보면서 주요한 함수, 코드를 확인하기 위해 타이핑한 용도로, 전체 소스코드가 아님에 주의해 주세요. 전체 코드는 교육 수강을 하면 완벽하게 받으실 수가 있답니다 ^^>
패스트캠퍼스 - 올인원 패키지 : 유니티 포트폴리오 완성 bit.ly/2R561g0
유니티 게임 포트폴리오 완성 올인원 패키지 Online. | 패스트캠퍼스
게임 콘텐츠 프로그래머로 취업하고 싶다면, 포트폴리오 완성은 필수! '디아블로'와 '배틀그라운드' 게임을 따라 만들어 보며, 프로그래머 면접에 나오는 핵심 개념까지 모두 잡아 보세요!
www.fastcampus.co.kr
'[컴퓨터] > 웹 | 앱 | 게임 개발' 카테고리의 다른 글
[패스트캠퍼스 수강 후기] 올인원 패키지 : 유니티 포트폴리오 완성 100% 환급 챌린지 37회차 미션 (0) | 2020.11.24 |
---|---|
[패스트캠퍼스 수강 후기] 올인원 패키지 : 유니티 포트폴리오 완성 100% 환급 챌린지 36회차 미션 (0) | 2020.11.23 |
[패스트캠퍼스 수강 후기] 올인원 패키지 : 유니티 포트폴리오 완성 100% 환급 챌린지 34회차 미션 (0) | 2020.11.21 |
[패스트캠퍼스 수강 후기] 올인원 패키지 : 유니티 포트폴리오 완성 100% 환급 챌린지 33회차 미션 (0) | 2020.11.20 |
[패스트캠퍼스 수강 후기] 올인원 패키지 : 유니티 포트폴리오 완성 100% 환급 챌린지 32회차 미션 (0) | 2020.11.19 |